

This method is a bit more Pythonic and allows us to skip the step of initializing an empty list. Now, let’s take a look at how we can accomplish this using a list comprehension. We then append the string to a new list to hold our hashed values. We can see here that we apply the same method as for a single string to each string in our list. Hashed_strings.append(hashlib.sha256(string.encode('utf-8')).hexdigest()) Let’s take a look at what the for loop method looks like first: # Hash a list of strings with hashlib.sha256 Let’s say that you have a list of strings that you need to hash, we can do this using either a for loop or a list comprehension. In many cases, you won’t just be working with a single string. Let’s see how we can take a unicode encoded string and return its HSA256 hash value using Python: # Hash a single string with hashlib.sha256 In this section, you’ll learn explicitly how to convert a unicode string to a SHA256 hash value.īecause the Python hashlib library cannot hash unicode encoded strings, such as those in utf-8, we need to first convert the string to bytes. In the above section, you learned how to use Python to implement a SHA256 hashing method. Want to learn more about Python for-loops? Check out my in-depth tutorial that takes your from beginner to advanced for-loops user! Want to watch a video instead? Check out my YouTube tutorial here. In the next section, we’ll explicitly cover off how to use Python SHA256 for unicode strings. Hashed_line = hashlib.sha256(line.rstrip()).hexdigest() Using the 'rb' method, we can write the following code, which would successfully hash a line: # Hash a file with hashlib.sha256 Now, if we were to try to hash the file using simply the 'r' method, it would raise a TypeError. In order to change this behaviour, we can change our context manager to the following: with open(file, 'rb') as f: The problem with this is that when we open a file using the 'r' method, Python implicitly asks Python to decode the bytes in the string to a default encoding, such as utf-8. You may be familiar with opening files using a context manager, such as the one shown below: with open(file, 'r') as f: Now, let’s take a look at how we can implement SHA256 for an entire file. In the above example, we first encoded the string and grabbed its hexadecimal value, passing it into the hash function. Hashed_string = hashlib.sha256(a_string.encode('utf-8')).hexdigest() Let’s take a look at how we can pass in a single string to return its hashed value using Python and hashlib: # Hash a single string with hashlib.sha256Ī_string = 'this string holds important and private information'
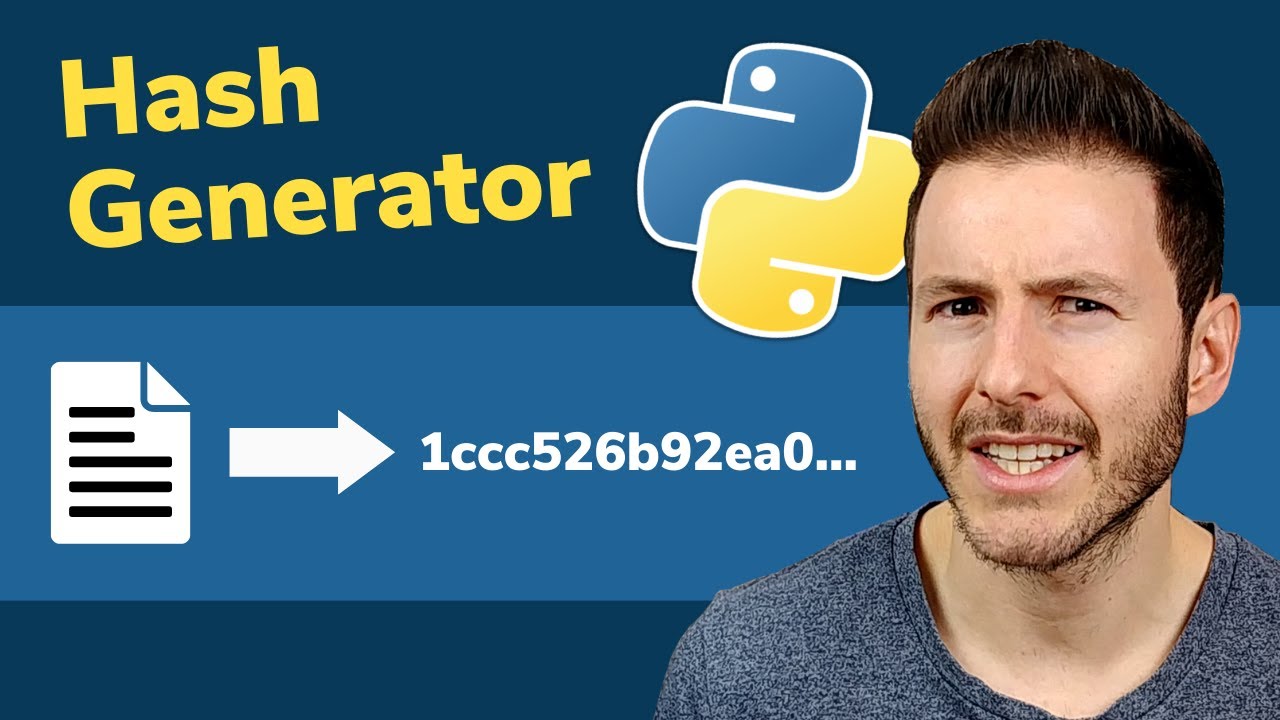
hexdigest() which is used to convert our data into hexadecimal format.encode() which is used to convert a string to bytes, meaning that the string can be passed into the sha256 function.


The sha256 constructor takes a byte-like input, returning a hashed value.The function has a number of associated with hashing values, which are especially useful given that normal strings can’t easily be processed: sha256() constructor is used to create a SHA256 hash. The module provides constructor methods for each type of hash. Python has a built-in library, hashlib, that is designed to provide a common interface to different secure hashing algorithms. In the next section, you’ll learn how to use the Python hashlib library to implement the SHA256 encryption algorithm.Ĭheck out some other Python tutorials on datagy, including our complete guide to styling Pandas and our comprehensive overview of Pivot Tables in Pandas! Using Python hashlib to Implement SHA256 This allows us to use unique identifiers, even when their data is obfuscated.
